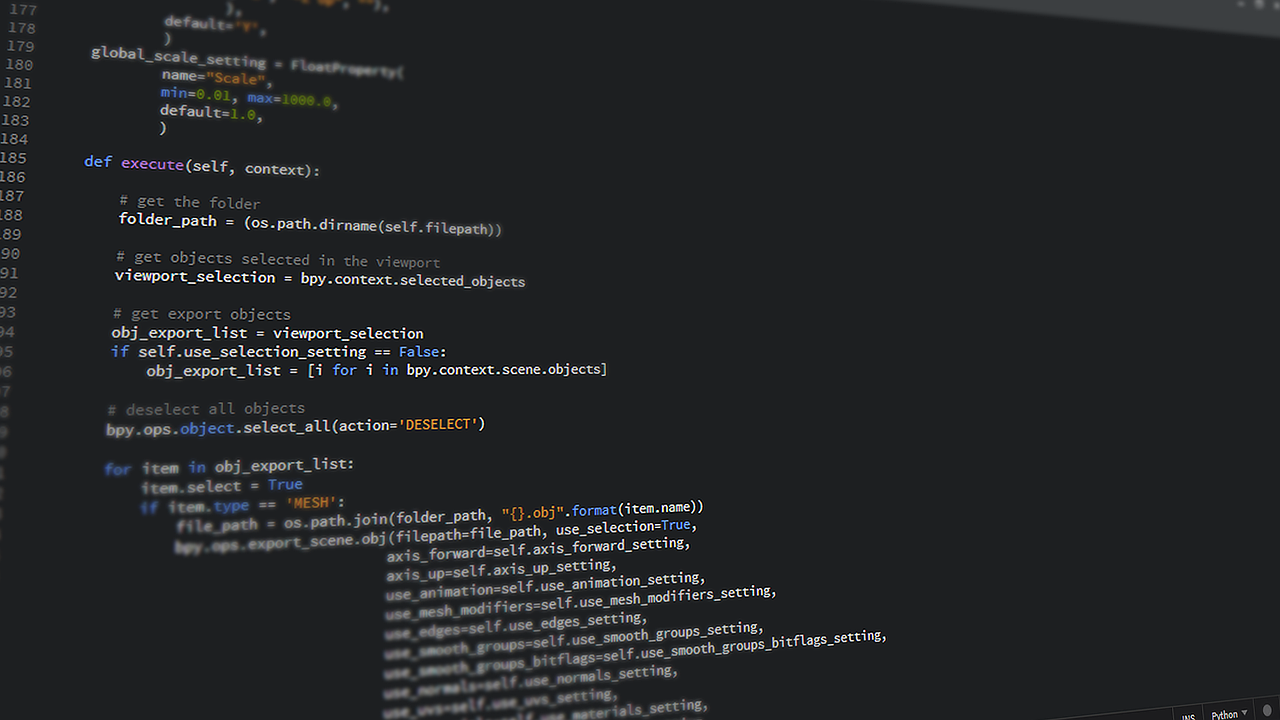
Practical Data Pipeline Performance Optimization with Python
In this follow-up blog post, we will explore practical examples of data pipeline performance optimizations using Python. We will cover parallel processing, data partitioning, caching, and other techniques to improve the efficiency of data pipelines.
Parallel Processing with Python
Multiprocessing
Python's built-in multiprocessing
library allows you to parallelize your code by utilizing multiple processes. Here's an example of using multiprocessing to calculate the square of a list of numbers:
import multiprocessing
def square(x):
return x * x
def main():
data = [1, 2, 3, 4, 5]
with multiprocessing.Pool() as pool:
results = pool.map(square, data)
print(results)
if __name__ == '__main__':
multiprocessing.freeze_support()
main()
Threading
Python's built-in threading
library enables you to parallelize your code using multiple threads. Note that the Global Interpreter Lock (GIL) in CPython can limit the performance benefits of multithreading for CPU-bound tasks. Here's an example using threading to download multiple web pages concurrently:
import requests
import threading
urls = ['https://www.pipelinemastery.io', 'https://www.unsplash.com']
def download(url):
response = requests.get(url)
return response.content
threads = [threading.Thread(target=download, args=(url,)) for url in urls]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
Data Partitioning with pandas
Using pandas, you can partition your data to improve processing efficiency. Here's an example of partitioning a DataFrame by date:
import pandas as pd
# Create a sample DataFrame with date and value columns
data = pd.DataFrame({'date': pd.date_range(start='2022-01-01', periods=10),
'value': range(10)})
# Partition the DataFrame by date
data['month'] = data['date'].dt.to_period('M')
partitions = data.groupby('month')
for month, partition in partitions:
print(f'Month: {month}')
print(partition)
Caching with Python
In-memory Caching
In-memory caching can significantly speed up data processing by storing frequently accessed data in memory. Here's an example using Python's built-in functools.lru_cache
to cache the results of a Fibonacci function:
import functools
@functools.lru_cache(maxsize=None)
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(100))
Distributed Caching
For distributed caching, you can use tools like Redis or Memcached. Here's an example using the redis
library to cache data in a Redis server:
import redis
cache = redis.StrictRedis(host='localhost', port=6379, db=0)
def get_data(key):
cached_data = cache.get(key)
if cached_data:
return cached_data.decode()
else:
data = f'Data for key {key}' # Replace with actual data retrieval
cache.set(key, data)
return data
print(get_data('example_key'))
Data Compression with Python
Data compression can help reduce storage and I/O costs. Here's an example of compressing and decompressing data using the gzip
library:
import gzip
data = b'Some data to be compressed'
# Compress the data
compressed_data = gzip.compress(data)
# Decompress the data
decompressed_data = gzip.decompress(compressed_data)
# Verify that the original data is recovered
assert data == decompressed_data
Efficient Data Loading with pandas
Loading data efficiently can improve the performance of data pipelines. Here's an example of loading a large CSV file in chunks using pandas:
import pandas as pd
def process_chunk(chunk):
# Perform data processing on the chunk here
pass
chunksize = 10 ** 5 # Adjust chunk size based on available memory
filename = 'large_dataset.csv'
# Read the CSV file in chunks
for chunk in pd.read_csv(filename, chunksize=chunksize):
process_chunk(chunk)
In this blog post, we've covered practical examples of data pipeline performance optimizations using Python, including parallel processing, data partitioning, caching, data compression, and efficient data loading. By applying these techniques in your data engineering projects, you can improve the efficiency and scalability of your data pipelines, reduce resource consumption, and enhance the overall performance of your data processing systems. Remember to continuously monitor and profile your data pipelines to identify and address performance bottlenecks and ensure optimal performance as your data processing requirements evolve over time.