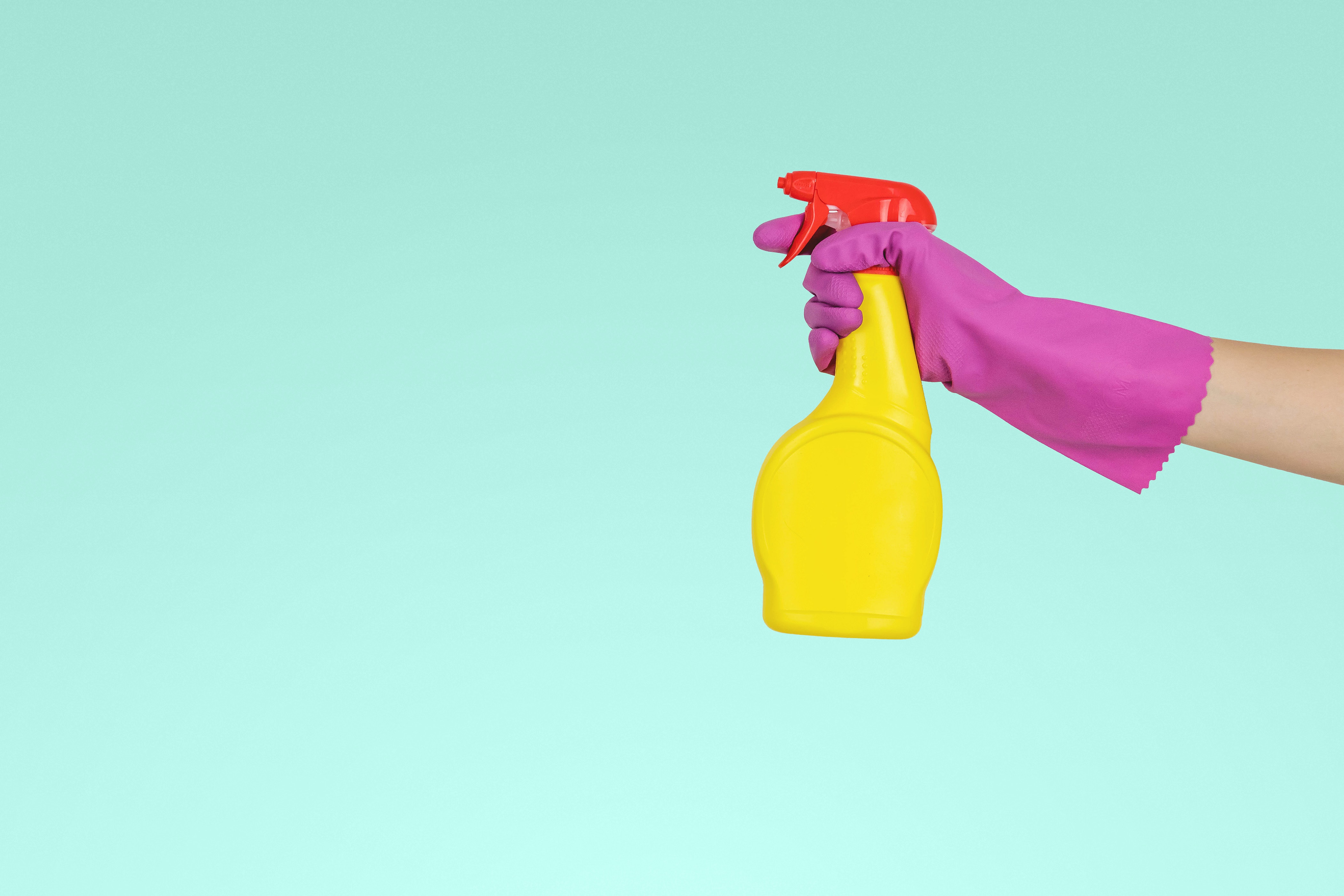
Data Transformation Techniques: A Comprehensive Guide for Data Engineers with Code Examples
Data transformation is a crucial process in data engineering, as it involves converting raw data into a structured and meaningful format suitable for analysis or further processing. This comprehensive guide covers various data transformation techniques, along with code examples in Python, to help data engineers build efficient and reliable data pipelines.
Data transformation involves a series of operations to convert raw data into a more structured and useful format. These operations include:
- Data cleaning
- Data normalization and standardization
- Data encoding and decoding
- Data aggregation and summarization
- Data reshaping and pivoting
Data Cleaning
Data cleaning involves identifying and correcting errors, inconsistencies, and inaccuracies in the data. Some common data cleaning techniques include:
- Removing duplicate records
- Filling missing values
- Correcting data entry errors
- Removing outliers
Example: Using Python and pandas to clean data:
CSV for the scripts to work:
id,gender,age,salary,job
1,M,25,50000,Engineer
2,F,32,55000,Engineer
3,M,29,60000,Data Analyst
4,F,35,65000,Data Scientist
5,M,42,70000,Data Scientist
6,F,38,75000,Manager
7,M,48,80000,Manager
8,F,45,85000,Executive
9,M,55,90000,Executive
10,F,50,95000,CEO
import pandas as pd
# Load data from CSV file
data = pd.read_csv('raw_data.csv')
# Remove duplicate records
data = data.drop_duplicates()
# Fill missing values using mean of the column
data['age'] = data['age'].fillna(data['age'].mean())
# Correct data entry errors (e.g., replace 'Male' with 'M' and 'Female' with 'F')
data['gender'] = data['gender'].replace({'Male': 'M', 'Female': 'F'})
# Remove outliers
Q1 = data['salary'].quantile(0.25)
Q3 = data['salary'].quantile(0.75)
IQR = Q3 - Q1
data = data[~((data['salary'] < (Q1 - 1.5 * IQR)) | (data['salary'] > (Q3 + 1.5 * IQR)))]
# Save cleaned data to a new CSV file
data.to_csv('cleaned_data.csv', index=False)
Data Normalization and Standardization
Data normalization and standardization involve scaling the data to a specific range or distribution to make it more suitable for analysis or modeling. Common techniques include:
- Min-max normalization
- Z-score standardization
Example: Using Python and scikit-learn to normalize and standardize data
import pandas as pd
from sklearn.preprocessing import MinMaxScaler, StandardScaler
# Load data from CSV file
data = pd.read_csv('cleaned_data.csv')
# Apply min-max normalization to age column
min_max_scaler = MinMaxScaler()
data['age_normalized'] = min_max_scaler.fit_transform(data[['age']])
# Apply z-score standardization to salary column
standard_scaler = StandardScaler()
data['salary_standardized'] = standard_scaler.fit_transform(data[['salary']])
# Save transformed data to a new CSV file
data.to_csv('transformed_data.csv', index=False)
Data Encoding and Decoding
Data encoding and decoding involve converting categorical data into numerical formats or vice versa. Common techniques include:
- Label encoding
- One-hot encoding
Example: Using Python and pandas to encode and decode categorical data
import pandas as pd
# Load data from CSV file
data = pd.read_csv('transformed_data.csv')
# Apply label encoding to gender column
data['gender_encoded'] = data['gender'].astype('category').cat.codes
# Apply one-hot encoding to the job column
data = pd.concat([data, pd.get_dummies(data['job'], prefix='job')], axis=1)
# Save encoded data to a new CSV file
data.to_csv('encoded_data.csv', index=False)
Data Aggregation and Summarization
Data aggregation and summarization involve combining and summarizing data to extract useful insights or reduce dimensionality. Common techniques include:
- Grouping and aggregating data
- Calculating summary statistics
Example: Using Python and pandas to aggregate and summarize data
import pandas as pd
# Load data from CSV file
data = pd.read_csv('encoded_data.csv')
# Group data by gender and calculate the average salary
average_salary_by_gender = data.groupby('gender')['salary'].mean().reset_index()
# Calculate summary statistics for the age column
age_summary = data['age'].describe()
# Save aggregated and summarized data to new CSV files
average_salary_by_gender.to_csv('average_salary_by_gender.csv', index=False)
age_summary.to_frame().to_csv('age_summary.csv')
Data Reshaping and Pivoting
Data reshaping and pivoting involve reorganizing the data structure to facilitate analysis or further processing. Common techniques include:
- Melting data from wide to long format
- Pivoting data from long to wide format
Example: Using Python and pandas to reshape and pivot data
import pandas as pd
# Load data from CSV file
data = pd.read_csv('encoded_data.csv')
# Melt data from wide to long format
melted_data = pd.melt(data, id_vars=['id', 'gender', 'age'], var_name='job', value_name='job_presence')
# Pivot data from long to wide format
pivoted_data = data.pivot_table(index=['id', 'gender', 'age'], columns='job', values='job_presence', fill_value=0)
# Save reshaped and pivoted data to new CSV files
melted_data.to_csv('melted_data.csv', index=False)
pivoted_data.reset_index().to_csv('pivoted_data.csv', index=False)
To finalize this post. Data transformation is a critical step in the data engineering process, and understanding various techniques can help you build efficient and reliable data pipelines. By leveraging Python and its powerful libraries like pandas and scikit-learn, you can easily implement data cleaning, normalization, standardization, encoding, decoding, aggregation, summarization, reshaping, and pivoting operations. Mastering these techniques will enable you to preprocess and transform your data effectively, making it more suitable for analysis, modeling, and further processing.
Β