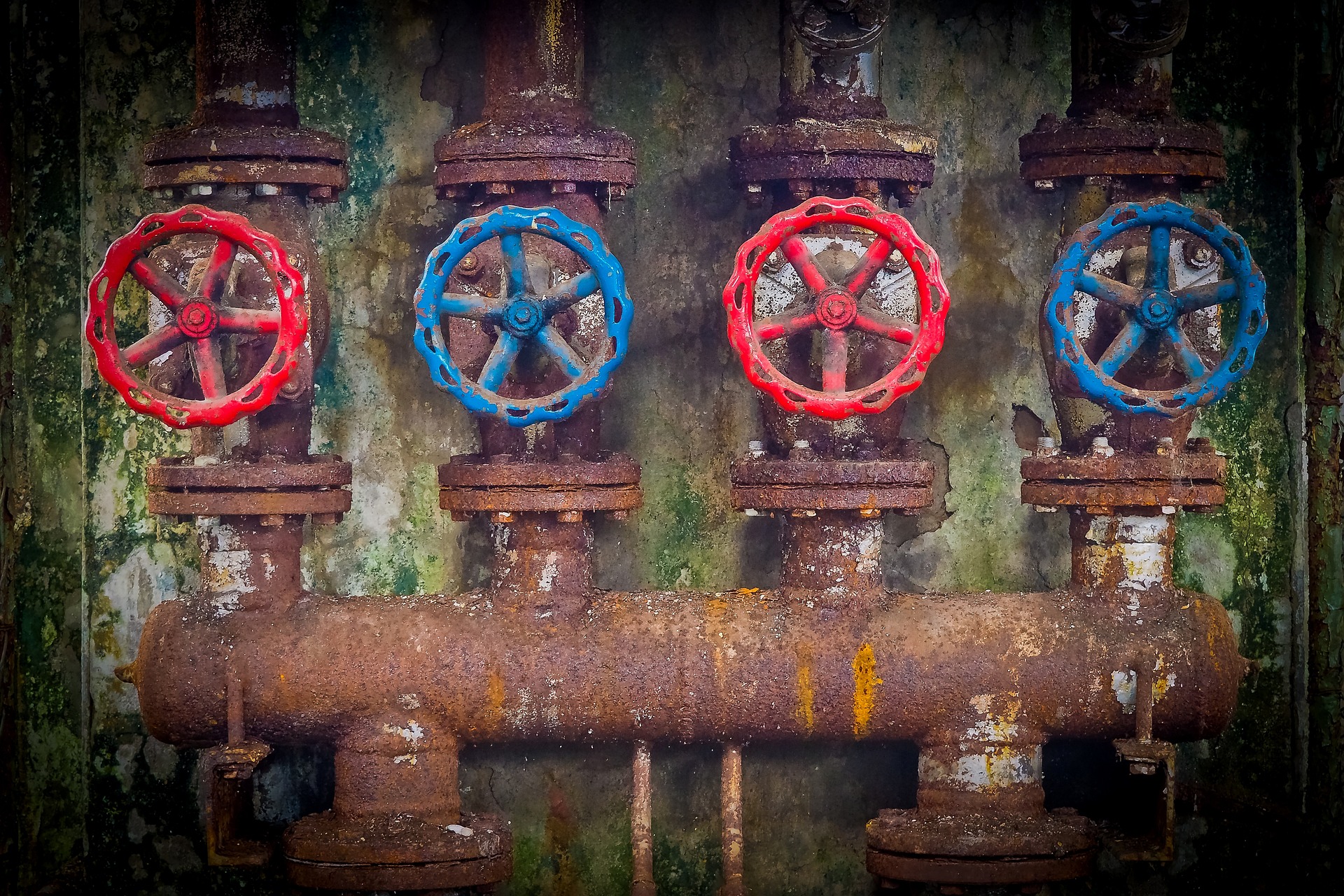
Data Pipelines 101: An Introduction for Data Engineers
Data pipelines are a critical aspect of data engineering, responsible for moving, transforming, and processing data from various sources to destinations. As the data-driven world continues to evolve, data engineers must develop robust data pipelines to ensure data flows smoothly and is readily available for analysis and decision-making. In this article, we'll provide a comprehensive introduction to data pipelines, covering their components, design principles, common tools and technologies, and a step-by-step example of building a simple data pipeline.
So... What is a Data Pipeline?
A data pipeline is a series of processes and tools that enable the flow of data from one system or component to another. Data pipelines are designed to collect, transform, and process data, ensuring that it is clean, accurate, and ready for analysis. Data pipelines should be able to handle a wide range of tasks, from simple data extraction and transformation to complex, multi-stage processing and machine learning workflows.
What are the Components of Data Pipeline?
A data pipeline typically consists of the following components:
- Data Ingestion: Which is the process of collecting data from various sources, such as databases, APIs, or files, and bringing it into the data pipeline for processing.
- Data Transformation: The process of converting raw data into a structured format that is suitable for analysis. This may involve cleaning, filtering, enriching, aggregating, or normalizing the data.
- Data Storage: The process of storing the transformed data in a suitable storage system, such as a data warehouse, data lake, or database, etc.
- Data Processing: The process of analyzing and processing the stored data to generate insights, reports, or predictions. This may involve querying the data using SQL, running machine learning algorithms, or applying statistical techniques.
- Data Consumption: The process of making the processed data available to end-users, such as business analysts, data scientists, or other applications. This may involve visualizing the data in dashboards or reports, exporting it to other systems, or integrating it with other applications.
Under what Principles should a Data Pipeline be Designed?
When designing a data pipeline, there are several key principles to consider:
- Scalability: The ability of the data pipeline to handle increasing data volumes and processing demands without impacting performance. This may involve scaling up the hardware resources or distributing the processing across multiple machines or clusters.
- Flexibility: The ability of the data pipeline to adapt to changing requirements, such as new data sources, formats, or processing tasks. This may involve using modular components, extensible frameworks, or configurable workflows.
- Reliability: The ability of the data pipeline to ensure data quality, consistency, and completeness. This may involve implementing data validation, error handling, and monitoring mechanisms.
- Maintainability: The ease with which the data pipeline can be maintained, debugged, and updated. This may involve using clear, well-documented code, modular components, and automated testing and deployment processes.
- Efficiency: The ability of the data pipeline to process and store data with minimal latency and resource consumption. This may involve optimizing the data storage format, algorithm, or hardware configuration.
What Use Cases can be Attributed to a Data Pipeline?
Data pipelines can be used in various scenarios, including:
- Data integration: Combining data from multiple sources, such as databases, APIs, or files, into a unified format for analysis. This may involve extracting, transforming, and loading (ETL) or extract, load, and transform (ELT) processes.
- Data warehousing: Collecting, transforming, and storing data from various sources in a central data warehouse for reporting and analysis.
- Real-time analytics: Processing and analyzing data in real-time or near-real-time to support operational decision-making and monitoring. This may involve using streaming data processing frameworks and techniques, such as Apache Kafka or Apache Flink.
- Machine learning: Building and deploying machine learning models that require large-scale data processing, feature engineering, and model training. Data pipelines can automate data preprocessing, model training, evaluation, and deployment.
- Data migration: Moving data between different storage systems, platforms, or formats, such as migrating data from on-premises databases to cloud-based data warehouses or from legacy systems to modern platforms.
- Data synchronization: Ensuring that data is consistent and up-to-date across multiple systems or applications, such as synchronizing data between databases, data lakes, or data warehouses.
What are Common Tools and Technologies for Data Pipelines?
There are various tools and technologies available for building data pipelines, ranging from open-source frameworks to commercial platforms. Some popular options include:
- Apache NiFi: An open-source data integration and ingestion platform that provides a web-based interface for designing, deploying, and monitoring data pipelines.
- Apache Airflow: An open-source platform for orchestrating complex data workflows, developed by Airbnb. Airflow allows you to define, schedule, and monitor data pipelines using Python code.
- Apache Kafka: A distributed streaming platform that can be used to build real-time data pipelines and streaming applications. Kafka provides high-throughput, fault-tolerant, and scalable data streaming capabilities.
- Apache Spark: A fast and general-purpose cluster-computing system for big data processing, with built-in modules for SQL, streaming, machine learning, and graph processing.
- Google Cloud Dataflow: A fully-managed service for building and executing data processing pipelines on the Google Cloud Platform. Dataflow provides auto-scaling, fault-tolerance, and real-time processing capabilities.
- AWS Glue: A fully-managed ETL service on the Amazon Web Services (AWS) platform. Glue allows you to discover, catalog, and transform data from various sources and load it into data warehouses, data lakes, or other storage systems.
- Microsoft Azure Data Factory: A cloud-based data integration service on the Microsoft Azure platform, allowing you to create, schedule, and manage data pipelines to move and transform data from various sources to destinations.
Code Example: Building a Simple Data Pipeline
To demonstrate the process of building a data pipeline, we'll create a simple example that reads a CSV file containing daily sales data, calculates the revenue for each row, and stores the results in an SQLite database.
We'll use Python and the pandas library for this example. First, make sure you have Python and pandas installed. Next, create a sample CSV file (daily_sales.csv) with the following data:
date,product_id,unit_price,quantity
2023-01-01,101,10.00,5
2023-01-01,102,20.00,3
2023-01-02,101,10.00,8
2023-01-02,103,30.00,2
import pandas as pd
import sqlite3
def read_csv(file_path):
return pd.read_csv(file_path)
def transform_data(data):
data['date'] = pd.to_datetime(data['date']).dt.date
data['revenue'] = data['unit_price'] * data['quantity']
return data
def load_data(data, db_file):
conn = sqlite3.connect(db_file)
data.to_sql('daily_sales', conn, if_exists='replace', index=False)
conn.close()
def main():
csv_file = 'daily_sales.csv'
db_file = 'sales_data.db'
raw_data = read_csv(csv_file)
transformed_data = transform_data(raw_data)
load_data(transformed_data, db_file)
if __name__ == '__main__':
main()
This script demonstrates the basic structure of a data pipeline, with separate functions for reading the CSV file, transforming the data, and loading it into an SQLite database. By running the script, you'll create an SQLite database file (sales_data.db) containing a table named "daily_sales" with the transformed data.
In summary, data pipelines play a crucial role in the data engineering process, enabling the flow of data between various systems and components. By understanding the core concepts, design principles, and technologies associated with data pipelines, you'll be well-equipped to build robust, scalable, and efficient data pipelines for a wide range of use cases.